The Tinify API allows you to compress and optimize JPEG and PNG images.It is designed as a REST service. The client libraries in variouslanguages make it very easy to interact with the Tinify API.
Installation
Creative photoshop youtube channel, Radhika Shimoga. Download and Install Imagenomic Noiseware 5.0.3 filter plugin for photoshop cc. Portraiture 3 FULL VERSION (mac 2018) + PROOF.
You can use the Java client as a Maven dependencyby adding the following to your application’s pom.xml
. To avoidbreaking changes in your code when we update the client, replaceRELEASE
with a specific version:
If you use Gradle instead, you can use the following:
The source code is available on Github.
Authentication
To use the API you must provide your API key. You canget an API key by registering with your name andemail address. Always keep your API key secret!
All requests will be made over an encryptedHTTPSconnection.
The giant kontakt library crack torrent. You can instruct the API client to make all requests over an HTTP proxy.Set the URL of your proxy server, which can optionally include credentials.
Compressing images
You can upload any JPEG or PNG image to the Tinify API to compress it. Wewill automatically detect the type of image and optimise with the TinyPNG orTinyJPG engine accordingly. Compression will start as soon as you upload afile or provide the URL to the image.
You can choose a local file as the source and write it to another file.
You can also upload an image from a buffer (a string with binary) and getthe compressed image data.
You can provide a URL to your image instead of having to upload it.
Resizing images
Use the API to create resized versions of your uploaded images. By lettingthe API handle resizing you avoid having to write such code yourself andyou will only have to upload your image once. The resized images will beoptimally compressed with a nice and crisp appearance.
You can also take advantage of intelligent cropping to create thumbnailsthat focus on the most visually important areas of your image.
Resizing counts as one additional compression. For example, if you uploada single image and retrieve the optimized version plus 2 resized versionsthis will count as 3 compressions in total.
To resize an image, call the resize
method on an image source:
The method
describes the way your image will be resized. The followingmethods are available:
scale
- Scales the image down proportionally. You must provide either a target
width
or a targetheight
, but not both. The scaled image will have exactly the provided width or height.
fit
- Scales the image down proportionally so that it fits within the given dimensions. You must provide both a
width
and aheight
. The scaled image will not exceed either of these dimensions.
cover
- Scales the image proportionally and crops it if necessary so that the result has exactly the given dimensions. You must provide both a
width
and aheight
. Which parts of the image are cropped away is determined automatically. An intelligent algorithm determines the most important areas of your image.
thumb
- A more advanced implementation of cover that also detects cut out images with plain backgrounds. The image is scaled down to the
width
andheight
you provide. If an image is detected with a free standing object it will add more background space where necessary or crop the unimportant parts. This feature is new and we’d love to hear your feedback!
If the target dimensions are larger than the original dimensions, the imagewill not be scaled up. Scaling up is prevented in order to protect thequality of your images.
Preserving metadata
You can request that specific metadata is copied from the uploaded imageto the compressed version. Preserving copyright
information, the GPSlocation
and the creation
date are currently supported. Preservingmetadata adds to the compressed file size, so you should only preservemetadata that is important to keep.
Preserving metadata will not count as an extra compression. However, inthe background the image will be created again with the additionalmetadata.
To preserve specific metadata, call the preserve
method on an imagesource:
You can provide the following options to preserve specific metadata. Nometadata will be added if the requested metadata is not present in theuploaded image.
copyright
- Preserves any copyright information. This includes the EXIF copyright tag (JPEG), the XMP rights tag (PNG) as well as a Photoshop copyright flag or URL. Uses up to 90 additional bytes, plus the length of the copyright data.
creation
- Preserves any creation date or time. This is the moment the image or photo was originally created. This includes the EXIF original date time tag (JPEG) or the XMP creation time (PNG). Uses around 70 additional bytes.
location
(JPEG only)- Preserves any GPS location data that describes where the image or photo was taken. This includes the EXIF GPS latitude and GPS longitude tags (JPEG). Uses around 130 additional bytes.
Saving to Amazon S3
You can tell the Tinify API to save compressed images directly to Amazon S3. If you useS3 to host your images this saves you the hassle of downloading images toyour server and uploading them to S3 yourself.
To save an image to S3, call the store
method on an image source:
You need to provide the following options in order to save an image onAmazon S3:
service
- Specify
s3
to store to Amazon S3. aws_access_key_id
aws_secret_access_key
- Your AWS access key ID and secret access key. These are the credentials to an Amazon AWS user account. Find out how to obtain them in Amazon’s documentation. The user must have the correct permissions, see below for details.
region
- The AWS region in which your S3 bucket is located.
path
- The path at which you want to store the image including the bucket name. The path must be supplied in the following format:
<bucket>/<path>/<filename>
.
The following settings are optional:
headers
(experimental)- You can add a
Cache-Control
header to control browser caching of the stored image, with for example:public, max-age=31536000
. The full list of directives can be found in the MDN web docs.
The user that corresponds to your AWS access key ID must have thePutObject
and PutObjectAcl
permissions on the paths of the objects youintend to create.
Example S3 access policy
If you want to create a user with limited access specifically for theTinify API, you can use the following example policyas a starting point:
Saving to Google Cloud Storage
You can tell the Tinify API to save compressed images directly to Google Cloud Storage.If you use GCS to host your images this saves you the hassle of downloadingimages to your server and uploading them to GCS yourself.
Before you can store an image in GCS you will need to generate an accesstoken with a service account.
We still need to create a piece of example code for this languagethat generates an access code. In case you have a working exampleready, please share your code!
Once you have generated the access token you can then save the optimisedimage directly to GCS by calling the store
method on an image source:
You need to provide the following options in order to save an image onGoogle Cloud Storage:
service
- Specify
gcs
to store to Google Cloud Storage. gcp_access_token
- The access token for authenticating to Google's Cloud Platform. Find out how to generate these tokens with the example above.
path
- The path at which you want to store the image including the bucket name. The path must be supplied in the following format:
<bucket>/<path>/<filename>
.
The following settings are optional:
headers
(experimental)- You can add a
Cache-Control
header to control browser caching of the stored image, with for example:public, max-age=31536000
. The full list of directives can be found in the MDN web docs.
Error handling
The Tinify API uses HTTP status codes to indicate success or failure. AnyHTTP errors are converted into exceptions, which are thrown by the clientlibrary.
There are four distinct types of errors. The exception message will containa more detailed description of the error condition.
AccountException
- There was a problem with your API key or with your API account. Yourrequest could not be authorized. If your compression limit is reached,you can wait until the next calendar month or upgrade your subscription. After verifying yourAPI key and your account status, you can retry the request.
ClientException
- The request could not be completed because of a problem with thesubmitted data. The exception message will contain more information.You should not retry the request.
ServerException
- The request could not be completed because of a temporary problem withthe Tinify API. It is safe to retry the request after a few minutes.If you see this error repeatedly for a longer period of time, pleasecontact us.
ConnectionException
- The request could not be sent because there was an issue connecting tothe Tinify API. You should verify your network connection. It is safe toretry the request.
You can handle each type of error separately:
If you are writing code that uses an API key configured by your users, youmay want to validate the API key before attempting to compress images.The validation makes a dummy request to check the network connection andverify the API key. An error is thrown if the dummy request fails.
Compression count
The API client automatically keeps track of the number of compressionsyou have made this month. You can get the compression count after youhave validated your API key or after you have made at least onecompression request.
Need help? Got feedback?
We’re always here to help, so if you’re stuck just drop us a noteon support@tinify.com. It’s alsothe perfect place to send us all your suggestions and feedback.
Adobe Photoshop CC 2019 v20.0 Free Download available for 32-bit and 64-bit operating system in our site you will get full standalone file setup in other words this is full offline installer. Furthermore, Setup file is working perfectly before uploading our team check all the files manually.
Review or description of Adobe Photoshop CC 2019 v20.0
Adobe Photoshop CC 2019 v20.0 is one of the powerful program for editing and modifying images. It is very popular application and widely used around the world for photo editing purpose. It is included wide variety of customization tools and features which provide full access to the digital photos. This application provides professional environment for designers, photographers, and artists. The interface of the Adobe Photoshop CC 2019 v20.0 is very straight forward and self-explaining which provide easy to perform for all the operations. It contains multiple capabilities of photo manipulation which allow users to enhanced the quality of images.
Furthermore, the application has got numerous sort of advanced features such as HDR images, manage colors, changes backgrounds, bundles of effects, histogram, brushes, layer control and multiple other. This latest version includes several enhancements in correction operations such as remove the chromatic aberrations, distortions and vignette. Customers also very handle pixel color, painting, scathing, and many other is very easy manners.
The most impressive feature of Adobe Photoshop CC is Mercury Graphic Engine which greatly improve the overall workflow and allow the customers to perform images editing without any trouble and difficulty. This powerful application contains many of the abilities for performing 3D designing in efficient way. All in a nutshell, we can say that it is one of the best photo editor program which includes bundles of advanced customization tools and options.
Features of Adobe Photoshop CC 2019 v20.0
There are some of the core features which user will experience after the installation of Adobe Photoshop CC 2019 v20.0.
- Professional application for editing your images
- It contains Bundles of capabilities for image manipulation without damaging the pixel quality
- You can very easily manage 30 million pixels and up to 3 GB of size through this application
- One of the popular graphics editor
- Adobe Photoshop CC has the ability for 3D designing
- The program capable for completing state-of-the-art digital images.
- Provide faster way for editing images and videos
- You can also manage the tone control using advanced features
- The program also got the capabilities for editing RAW images formats
- Includes hundreds of effects which enhanced the quality of your images
- Provide faster response to the users as compared to other related applications
- The program got black and white conversion abilities which provide color your images via huge collections of presets
- You can very easily do multiple operations like resize the image, cropping, rotating and etc.
- Users also work with vector graphics
- It supports almost all the images formats as well as adobe illustrator formats
Technical Details of Adobe Photoshop CC 2019 v20.0
- Size of Setup File: 1.93 GB, 200MB
- Setup Type: Offline Installer
- Well-suited with: 32-Bit (x86) / 64-Bit (64)
- Added Date of Latest Version: 09 Dec 2018
System requirements of Adobe Photoshop CC 2019 v20.0 Free Download
Before starting download Adobe Photoshop CC 2019 v20.0 your system must fulfill these requirements to use this program.
- Supporting windows: 10 / 8.1 / 8 / 7
- Hard Disk Space: 6 GB
- RAM Memory: 2 GB required or above
- Processor: Intel Dual Core Processor or above
How to Install This Software
- Firstly, you can unzip file using WinRAR or any other software.
- Secondly you open the setup and accept the terms and clink on the install button.
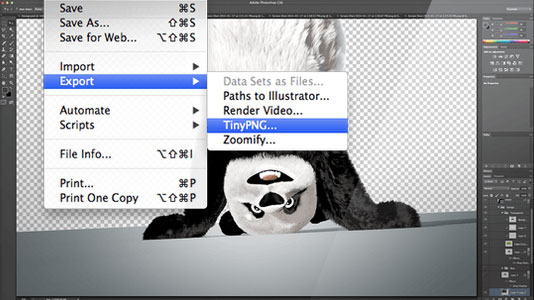
Adobe Photoshop CC 2019 v20.0 Free Download
Click on the below button downloading for the Adobe Photoshop CC 2019 v20.0. This is full offline installer and standalone setup for 64-bit operating system. You can also download Adobe Photoshop CC 2018 v19.1 plus portable.